Objectives
- Apply knowledge of polymorphism to a practical project scenario.
- Apply knowledge of encapsulation to a practical project scenario.
- Apply knowledge of inheritance to a practical project scenario.
- Create new classes to instantiate instances of objects from.
- Generate web controls at runtime.
- Demonstrate ability to appropriately create and use random numbers.
Background
Sudoku is the addictive number puzzle that has taken the UK by storm and is now taking over the rest of the world. Since it first appeared in The Times in late 2004 its popularity has grow so that it appears in most UK newspapers and has spread to countries from India to Canada, Australia to South Africa. Sudoku first appeared in a US puzzle magazine in the late 1970s, but was then picked up by Japanese publisher Nikoli who dubbed it Su Doku or ‘Solitary Square’ and the puzzle-hungry Japanese loved it. From there it was discovered by The Times and the rest is history.
Sudoku is played on a nine by nine grid consisting of 81 total squares. The object of the puzzle is to fill in the digits from 1 to 9 so that each digit appears only once in each column, row and small three by three square (see Figure 1). You are given some of the numbers and you have to use logic and deduction to find the position of the other numbers.
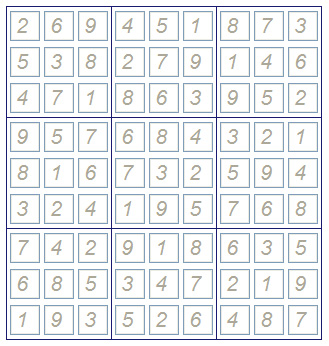
Figure 1. A solved Sudoku puzzle.
You must create an ASP.NET version of Sudoku similar to this version. Here is another version to look at: version 2. Here is a third version to look at: version 3. This server only holds the aspx file. There is no source code on this server.
Requirements
While the structure of this project is provided below, it is up to you to implement the functionality of the project using the structure provided.
- Inheritance
- Inheritance allows code reuse by building on existing classes. It is the concept of deriving classes from a base class where the derived class "is a" one of the base class.
- Box must inherit from BaseBox
- Puzzle must inherit from BaseBox
- Polymorphism
- In C#, polymorphism means the ability for classes to share the same methods (actions) but implement them differently.
- BaseBox, Box, Puzzle all have different implementations of getItem, setItem, getItemValue
- Calling BaseBox.setItem() has a different implementation from Box.setItem()
- Encapsulation
- Encapsulation is a method of hiding object data and implementation details, but defining properties and methods to let the caller have access to it.
- Box, Puzzle, SudokuTextBox, Number all have private variables / objects
- The only way to access these is via get and set methods
- BaseBox.cs (BaseBox class)
- Base class to be inherited - it is an abstract class
- Constructor
- Definition for getItem()
- So that child classes will inherit this function
- Definition for setItem()
- So that child classes will inherit this function
- Definition for getItemValue()
- So that child classes will inherit this function
- Box.cs (Box class)
- Represents a single 3x3 Box
- Inherits from BaseBox
- Each instance holds a private array of SudokuTextBox
- Constructor
- instantiates a new 3x3 SudokuTextBox
- getItem(int row, int col)
- polymorphism & encapsulation: returns a SudokuTextBox
- setItem(SudokuTextBox oTb, int row, int col)
- polymorphism & encapsulation: sets the instance of SudokuTextBox equal to what is passed in
- getItemValue(int row, int col)
- polymorphism & encapsulation: overwrites BaseBox.getItemValue and returns the value of SudokuTextBox[row,col]
- Puzzle.cs (Puzzle class)
- Represents the entire puzzle, containing 9 instances of Box
- Inherits from BaseBox
- Each instance holds a private array of Box
- Constructor
- instantiates a new 3x3 Box (the Box class above)
- getItem(int row, int col)
- polymorphism & encapsulation: returns an instance of Box
- setItem(Box oBox, int row, int col)
- polymorphism & encapsulation: Sets an array index of puzzle equal to the Box passed in
- getItemValue(int row, int col)
- polymorphism & encapsulation: returns the string value of an array index of puzzle
- SudokuTextBox.cs (SudokuTextBox class)
- Contains a private instance of System.Web.UI.WebControls.TextBox
- Contains a private boolean visibility variable / operator
- Contains a private instance of Number
- Constructor initializes the TextBox, Number, and visibility
- getTextBox()
- returns an instance of System.Web.UI.WebControls.TextBox
- getTextBoxValue()
- returns a Number object (instance of the Number class)
- setTextBoxValue(Number nmbr)
- getVisibility()
- setVisibility()
- setWrongNumber()
- setCorrectNumber()
- setHint()
- Number.cs (Number class)
- Contains a private integer
- Constructor
- initialized the number to 0
- getNumber()
- returns the number as an integer
- setNumber(int number)
- sudoku.aspx
- Do not create elements for textboxes here. Simply place a Panel here, along with other aesthetics for your project.
- Puzzle
- Solution Button (code for functionality belongs in the CodeBehind)
- Shows the completed Sudoku puzzle, disabled.
- New Puzzle Button (code for functionality belongs in the CodeBehind)
- Generates a new puzzle to start over with.
- Check It Button (code for functionality belongs in the CodeBehind)
- Checks each TextBox to determine if the value entered is the correct Number
- Highlights the cell if it is incorrect.
- Help / Hint Button (code for functionality belongs in the CodeBehind)
- Randomly adds another disabled TextBox to the puzzle, increasing the number displayed by the program by 1.
- Each help/hint TextBox should be highlighted, but highlighted differently from the Check It functionality so that the two can be distinguished from one another.
- Links for Easy, Medium, Hard
- Easy is defined for this project as:
- 32 numbers displayed at random
- No more than 4 numbers per box displayed
- No more than 4 occurances of each number displayed
- Medium is defined for this project as:
- 30 numbers displayed at random
- No more than 4 numbers per box displayed
- No more than 5 occurances of each number displayed
- Hard is defined for this project as:
- 28 numbers displayed at random
- No more than 5 numbers per box displayed
- No more than 5 occurances of each number displayed
- sudoku.aspx.cs
- Variables for the solution
- Solutions stored as multi-dimensional arrays
- Provide 10 different solutions
- Use a random number generator to determine which of the 10 gets displayed each time the page is navigated to.
- Input validation
- If something other than a number is entered, either (a) mark it as incorrect when Check It is clicked, (b) remove it from the puzzle when Check It is clicked, or (c) do not allow it to be entered in the first place.
- Page_Load()
- Perform page initialization here. Instantiate the necessary objects and call the necessary functions to load your puzzle.
- Generate Sudoku puzzle
- Create the necessary instances of Box, SudokuTextBox, and Number
- Populate the instances with the correct number pulled from the solution array
- Build Sudoku table by using Table, TableRow, and TableCell
Turn In
- sudoku.aspx: All C# code is contained in the Code Behind
- sudoku.aspx.cs
- Box.cs
- Puzzle.cs
- SudokuTextBox.cs
- Number.cs
- BaseBox.cs
- Other associated project files
Above & Beyond
- Create a second version (in addition to the above version - do not replace the above version and do not replace/alter any of the code for the above version because the above version will be graded against the requirements above) that displays the initial numbers by following a pattern instead of displaying them at random.
Create 5 different patterns that can be implemented and randomly choose which pattern will be implemented upon page load. The pattern should exist such that it is symmetric when the puzzle is folded in half on the horizontal (see Figure 2).
Figure 2. Sudoku puzzle split in half horizontally to show symmetry between the top and bottom halves.
Grading
If the project is not functional (does not work), then your project grade starts at 50%. This is a professional project; our company will not accept partial work. Late work will not be accepted. (In the real world, you would not get paid for a project you did not complete. In our world, you start at 50%)
- 20% Aesthetics & Usability
- (Professional, effective and easy navigation, common scheme across
entire site, use of images, originality, creativity, usability features, intuitive, user friendly, easy to use, etc. -- More than just form elements -- CSS, look 'n' feel, etc.)
- 55% Coding, Logic, & Functionality
- (Does not crash, efficiency of code, proper indentation, neatness, free from errors, correct logic, security, follows typical coding standards, apparent planning, etc.)
- 15% Commenting
- (In general, one line of comment per line of code)
- 10% Above & Beyond
- The minimum requirements for an A on a perfect project are completion of the requirements above. The second version listed under Above & Beyond must be successfully completed in order to achieve 100% on a perfect project.
CGT 456
|